|
| 1 | +# 1609. Even Odd Tree |
| 2 | + |
| 3 | +- Difficulty: Medium. |
| 4 | +- Related Topics: Tree, Breadth-First Search, Binary Tree. |
| 5 | +- Similar Questions: . |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +A binary tree is named **Even-Odd** if it meets the following conditions: |
| 10 | + |
| 11 | + |
| 12 | + |
| 13 | +- The root of the binary tree is at level index `0`, its children are at level index `1`, their children are at level index `2`, etc. |
| 14 | + |
| 15 | +- For every **even-indexed** level, all nodes at the level have **odd** integer values in **strictly increasing** order (from left to right). |
| 16 | + |
| 17 | +- For every **odd-indexed** level, all nodes at the level have **even** integer values in **strictly decreasing** order (from left to right). |
| 18 | + |
| 19 | + |
| 20 | +Given the `root` of a binary tree, **return **`true`** if the binary tree is **Even-Odd**, otherwise return **`false`**.** |
| 21 | + |
| 22 | + |
| 23 | +Example 1: |
| 24 | + |
| 25 | +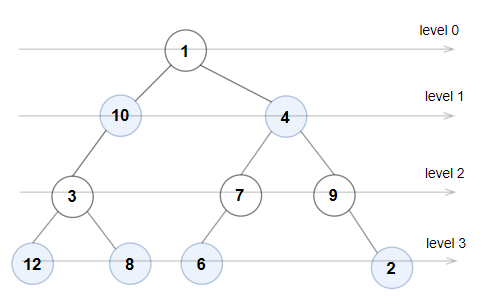 |
| 26 | + |
| 27 | +``` |
| 28 | +Input: root = [1,10,4,3,null,7,9,12,8,6,null,null,2] |
| 29 | +Output: true |
| 30 | +Explanation: The node values on each level are: |
| 31 | +Level 0: [1] |
| 32 | +Level 1: [10,4] |
| 33 | +Level 2: [3,7,9] |
| 34 | +Level 3: [12,8,6,2] |
| 35 | +Since levels 0 and 2 are all odd and increasing and levels 1 and 3 are all even and decreasing, the tree is Even-Odd. |
| 36 | +``` |
| 37 | + |
| 38 | +Example 2: |
| 39 | + |
| 40 | +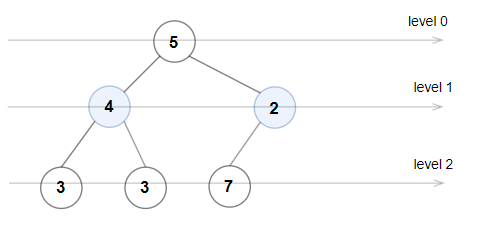 |
| 41 | + |
| 42 | +``` |
| 43 | +Input: root = [5,4,2,3,3,7] |
| 44 | +Output: false |
| 45 | +Explanation: The node values on each level are: |
| 46 | +Level 0: [5] |
| 47 | +Level 1: [4,2] |
| 48 | +Level 2: [3,3,7] |
| 49 | +Node values in level 2 must be in strictly increasing order, so the tree is not Even-Odd. |
| 50 | +``` |
| 51 | + |
| 52 | +Example 3: |
| 53 | + |
| 54 | +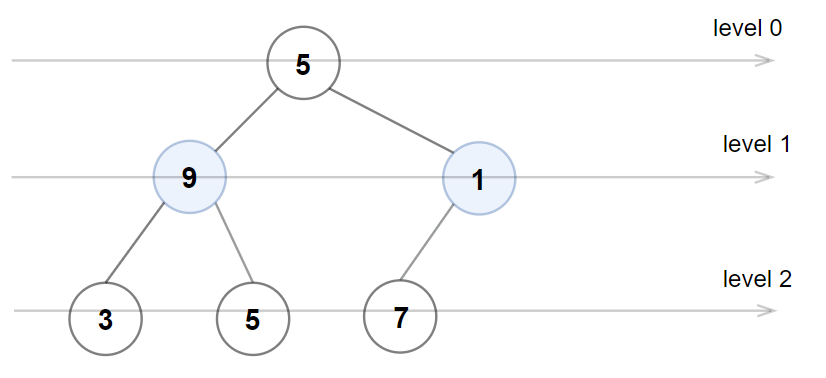 |
| 55 | + |
| 56 | +``` |
| 57 | +Input: root = [5,9,1,3,5,7] |
| 58 | +Output: false |
| 59 | +Explanation: Node values in the level 1 should be even integers. |
| 60 | +``` |
| 61 | + |
| 62 | + |
| 63 | +**Constraints:** |
| 64 | + |
| 65 | + |
| 66 | + |
| 67 | +- The number of nodes in the tree is in the range `[1, 105]`. |
| 68 | + |
| 69 | +- `1 <= Node.val <= 106` |
| 70 | + |
| 71 | + |
| 72 | + |
| 73 | +## Solution |
| 74 | + |
| 75 | +```javascript |
| 76 | +/** |
| 77 | + * Definition for a binary tree node. |
| 78 | + * function TreeNode(val, left, right) { |
| 79 | + * this.val = (val===undefined ? 0 : val) |
| 80 | + * this.left = (left===undefined ? null : left) |
| 81 | + * this.right = (right===undefined ? null : right) |
| 82 | + * } |
| 83 | + */ |
| 84 | +/** |
| 85 | + * @param {TreeNode} root |
| 86 | + * @return {boolean} |
| 87 | + */ |
| 88 | +var isEvenOddTree = function(root) { |
| 89 | + var nodesOfLevel = [root]; |
| 90 | + var level = 0; |
| 91 | + while (nodesOfLevel.length) { |
| 92 | + var newNodesOfLevel = []; |
| 93 | + for (var i = 0; i < nodesOfLevel.length; i++) { |
| 94 | + if (level % 2) { |
| 95 | + if (nodesOfLevel[i].val % 2) return false; |
| 96 | + if (i > 0 && nodesOfLevel[i].val >= nodesOfLevel[i - 1].val) return false; |
| 97 | + } else { |
| 98 | + if (nodesOfLevel[i].val % 2 === 0) return false; |
| 99 | + if (i > 0 && nodesOfLevel[i].val <= nodesOfLevel[i - 1].val) return false; |
| 100 | + } |
| 101 | + nodesOfLevel[i].left && newNodesOfLevel.push(nodesOfLevel[i].left); |
| 102 | + nodesOfLevel[i].right && newNodesOfLevel.push(nodesOfLevel[i].right); |
| 103 | + } |
| 104 | + nodesOfLevel = newNodesOfLevel; |
| 105 | + level += 1; |
| 106 | + } |
| 107 | + return true; |
| 108 | +}; |
| 109 | +``` |
| 110 | + |
| 111 | +**Explain:** |
| 112 | + |
| 113 | +nope. |
| 114 | + |
| 115 | +**Complexity:** |
| 116 | + |
| 117 | +* Time complexity : O(n). |
| 118 | +* Space complexity : O(n). |
0 commit comments