|
| 1 | +# 1642. Furthest Building You Can Reach |
| 2 | + |
| 3 | +- Difficulty: Medium. |
| 4 | +- Related Topics: Array, Greedy, Heap (Priority Queue). |
| 5 | +- Similar Questions: Make the Prefix Sum Non-negative, Find Building Where Alice and Bob Can Meet. |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +You are given an integer array `heights` representing the heights of buildings, some `bricks`, and some `ladders`. |
| 10 | + |
| 11 | +You start your journey from building `0` and move to the next building by possibly using bricks or ladders. |
| 12 | + |
| 13 | +While moving from building `i` to building `i+1` (**0-indexed**), |
| 14 | + |
| 15 | + |
| 16 | + |
| 17 | +- If the current building's height is **greater than or equal** to the next building's height, you do **not** need a ladder or bricks. |
| 18 | + |
| 19 | +- If the current building's height is **less than** the next building's height, you can either use **one ladder** or `(h[i+1] - h[i])` **bricks**. |
| 20 | + |
| 21 | + |
| 22 | +**Return the furthest building index (0-indexed) you can reach if you use the given ladders and bricks optimally.** |
| 23 | + |
| 24 | + |
| 25 | +Example 1: |
| 26 | + |
| 27 | +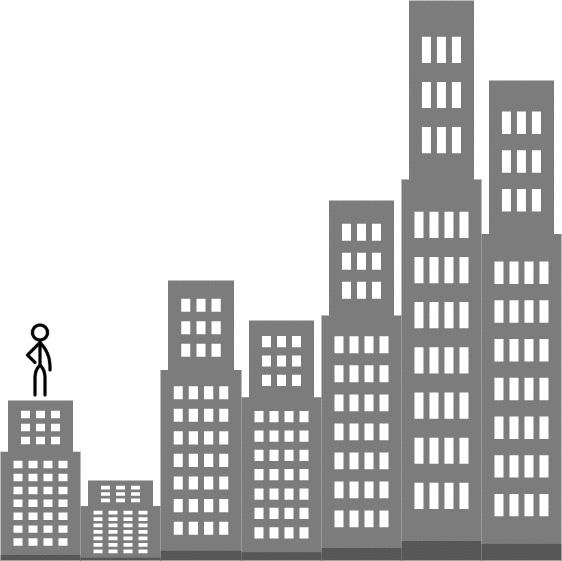 |
| 28 | + |
| 29 | +``` |
| 30 | +Input: heights = [4,2,7,6,9,14,12], bricks = 5, ladders = 1 |
| 31 | +Output: 4 |
| 32 | +Explanation: Starting at building 0, you can follow these steps: |
| 33 | +- Go to building 1 without using ladders nor bricks since 4 >= 2. |
| 34 | +- Go to building 2 using 5 bricks. You must use either bricks or ladders because 2 < 7. |
| 35 | +- Go to building 3 without using ladders nor bricks since 7 >= 6. |
| 36 | +- Go to building 4 using your only ladder. You must use either bricks or ladders because 6 < 9. |
| 37 | +It is impossible to go beyond building 4 because you do not have any more bricks or ladders. |
| 38 | +``` |
| 39 | + |
| 40 | +Example 2: |
| 41 | + |
| 42 | +``` |
| 43 | +Input: heights = [4,12,2,7,3,18,20,3,19], bricks = 10, ladders = 2 |
| 44 | +Output: 7 |
| 45 | +``` |
| 46 | + |
| 47 | +Example 3: |
| 48 | + |
| 49 | +``` |
| 50 | +Input: heights = [14,3,19,3], bricks = 17, ladders = 0 |
| 51 | +Output: 3 |
| 52 | +``` |
| 53 | + |
| 54 | + |
| 55 | +**Constraints:** |
| 56 | + |
| 57 | + |
| 58 | + |
| 59 | +- `1 <= heights.length <= 105` |
| 60 | + |
| 61 | +- `1 <= heights[i] <= 106` |
| 62 | + |
| 63 | +- `0 <= bricks <= 109` |
| 64 | + |
| 65 | +- `0 <= ladders <= heights.length` |
| 66 | + |
| 67 | + |
| 68 | + |
| 69 | +## Solution |
| 70 | + |
| 71 | +```javascript |
| 72 | +/** |
| 73 | + * @param {number[]} heights |
| 74 | + * @param {number} bricks |
| 75 | + * @param {number} ladders |
| 76 | + * @return {number} |
| 77 | + */ |
| 78 | +var furthestBuilding = function(heights, bricks, ladders) { |
| 79 | + var queue = new MinPriorityQueue(); |
| 80 | + for (var i = 0; i < heights.length - 1; i++) { |
| 81 | + if (heights[i + 1] <= heights[i]) continue; |
| 82 | + queue.enqueue(heights[i + 1] - heights[i], heights[i + 1] - heights[i]); |
| 83 | + if (queue.size() > ladders) { |
| 84 | + var height = queue.dequeue().element; |
| 85 | + if (bricks < height) return i; |
| 86 | + bricks -= height; |
| 87 | + } |
| 88 | + } |
| 89 | + return heights.length - 1; |
| 90 | +}; |
| 91 | +``` |
| 92 | + |
| 93 | +**Explain:** |
| 94 | + |
| 95 | +nope. |
| 96 | + |
| 97 | +**Complexity:** |
| 98 | + |
| 99 | +* Time complexity : O(n * log(n)). |
| 100 | +* Space complexity : O(n). |
0 commit comments