Commit a58d06d
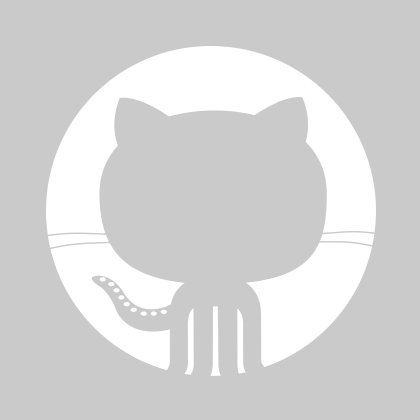
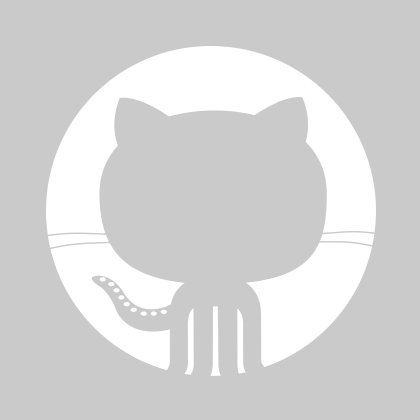
Shun Fan
Jon Wayne Parrott
1 parent 03251f8 commit a58d06d
File tree
4 files changed
+142
-75
lines changed- storage/transfer_service
4 files changed
+142
-75
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
11 | 11 |
| |
12 | 12 |
| |
13 | 13 |
| |
14 |
| - | |
| 14 | + | |
15 | 15 |
| |
16 | 16 |
| |
17 | 17 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| 15 | + | |
| 16 | + | |
15 | 17 |
| |
16 | 18 |
| |
17 | 19 |
| |
| |||
22 | 24 |
| |
23 | 25 |
| |
24 | 26 |
| |
25 |
| - | |
26 |
| - | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
27 | 31 |
| |
28 | 32 |
| |
29 | 33 |
| |
30 | 34 |
| |
31 | 35 |
| |
32 | 36 |
| |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
43 | 46 |
| |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
48 | 51 |
| |
49 |
| - | |
50 |
| - | |
51 |
| - | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
52 | 55 |
| |
53 | 56 |
| |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
59 |
| - | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
60 | 63 |
| |
61 | 64 |
| |
62 |
| - | |
63 |
| - | |
| 65 | + | |
| 66 | + | |
64 | 67 |
| |
65 | 68 |
| |
66 | 69 |
| |
67 |
| - | |
68 | 70 |
| |
69 |
| - | |
70 |
| - | |
| 71 | + | |
| 72 | + | |
71 | 73 |
| |
| 74 | + | |
72 | 75 |
| |
73 | 76 |
| |
74 |
| - | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
75 | 107 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| 15 | + | |
| 16 | + | |
15 | 17 |
| |
16 | 18 |
| |
17 | 19 |
| |
| |||
22 | 24 |
| |
23 | 25 |
| |
24 | 26 |
| |
25 |
| - | |
26 |
| - | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
27 | 32 |
| |
28 | 33 |
| |
29 | 34 |
| |
30 | 35 |
| |
31 | 36 |
| |
32 | 37 |
| |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
43 | 47 |
| |
44 |
| - | |
45 |
| - | |
46 |
| - | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
47 | 51 |
| |
48 | 52 |
| |
49 |
| - | |
50 |
| - | |
51 |
| - | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
52 | 56 |
| |
53 |
| - | |
54 |
| - | |
| 57 | + | |
| 58 | + | |
55 | 59 |
| |
56 |
| - | |
57 |
| - | |
| 60 | + | |
| 61 | + | |
58 | 62 |
| |
59 |
| - | |
60 |
| - | |
| 63 | + | |
| 64 | + | |
61 | 65 |
| |
62 | 66 |
| |
63 | 67 |
| |
64 |
| - | |
65 |
| - | |
66 |
| - | |
| 68 | + | |
| 69 | + | |
67 | 70 |
| |
| 71 | + | |
68 | 72 |
| |
69 | 73 |
| |
70 |
| - | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
71 | 99 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| 15 | + | |
15 | 16 |
| |
16 | 17 |
| |
17 | 18 |
| |
| |||
21 | 22 |
| |
22 | 23 |
| |
23 | 24 |
| |
24 |
| - | |
25 |
| - | |
26 |
| - | |
27 | 25 |
| |
28 |
| - | |
29 |
| - | |
| 26 | + | |
| 27 | + | |
30 | 28 |
| |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
31 | 33 |
| |
32 | 34 |
| |
33 | 35 |
| |
34 | 36 |
| |
35 |
| - | |
| 37 | + | |
| 38 | + | |
36 | 39 |
| |
37 | 40 |
| |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 | 41 |
| |
47 | 42 |
| |
| 43 | + | |
48 | 44 |
| |
49 | 45 |
| |
50 |
| - | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
51 | 58 |
|
0 commit comments