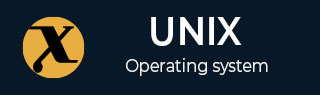
- Unix / Linux - Home
- Unix / Linux - What is Linux?
- Unix / Linux - Getting Started
- Unix / Linux - File Management
- Unix / Linux - Directories
- Unix / Linux - File Permission
- Unix / Linux - Environment
- Unix / Linux - Basic Utilities
- Unix / Linux - Pipes & Filters
- Unix / Linux - Processes
- Unix / Linux - Communication
- Unix / Linux - The vi Editor
- Unix / Linux - Shell Scripting
- Unix / Linux - What is Shell?
- Unix / Linux - Using Variables
- Unix / Linux - Special Variables
- Unix / Linux - Using Arrays
- Unix / Linux - Basic Operators
- Unix / Linux - Decision Making
- Unix / Linux - Shell Loops
- Unix / Linux - Loop Control
- Unix / Linux - Shell Substitutions
- Unix / Linux - Quoting Mechanisms
- Unix / Linux - IO Redirections
- Unix / Linux - Shell Functions
- Unix / Linux - Manpage Help
- Advanced Unix / Linux
- Unix / Linux - Standard I/O Streams
- Unix / Linux - File Links
- Unix / Linux - Regular Expressions
- Unix / Linux - File System Basics
- Unix / Linux - User Administration
- Unix / Linux - System Performance
- Unix / Linux - System Logging
- Unix / Linux - Signals and Traps
Unix / Linux - Shell Relational Operators Example
Bourne Shell supports the following relational operators that are specific to numeric values. These operators do not work for string values unless their value is numeric.
Assume variable a holds 10 and variable b holds 20 then −
Operator | Description | Example |
---|---|---|
-eq | Checks if the value of two operands are equal or not; if yes, then the condition becomes true. | [ $a -eq $b ] is not true. |
-ne | Checks if the value of two operands are equal or not; if values are not equal, then the condition becomes true. | [ $a -ne $b ] is true. |
-gt | Checks if the value of left operand is greater than the value of right operand; if yes, then the condition becomes true. | [ $a -gt $b ] is not true. |
-lt | Checks if the value of left operand is less than the value of right operand; if yes, then the condition becomes true. | [ $a -lt $b ] is true. |
-ge | Checks if the value of left operand is greater than or equal to the value of right operand; if yes, then the condition becomes true. | [ $a -ge $b ] is not true. |
-le | Checks if the value of left operand is less than or equal to the value of right operand; if yes, then the condition becomes true. | [ $a -le $b ] is true. |
It is very important to understand that all the conditional expressions should be placed inside square braces with spaces around them. For example, [ $a <= $b ] is correct whereas, [$a <= $b] is incorrect.
Example
Here is an example which uses all the relational operators −
#!/bin/sh a=10 b=20 if [ $a -eq $b ] then echo "$a -eq $b : a is equal to b" else echo "$a -eq $b: a is not equal to b" fi if [ $a -ne $b ] then echo "$a -ne $b: a is not equal to b" else echo "$a -ne $b : a is equal to b" fi if [ $a -gt $b ] then echo "$a -gt $b: a is greater than b" else echo "$a -gt $b: a is not greater than b" fi if [ $a -lt $b ] then echo "$a -lt $b: a is less than b" else echo "$a -lt $b: a is not less than b" fi if [ $a -ge $b ] then echo "$a -ge $b: a is greater or equal to b" else echo "$a -ge $b: a is not greater or equal to b" fi if [ $a -le $b ] then echo "$a -le $b: a is less or equal to b" else echo "$a -le $b: a is not less or equal to b" fi
The above script will generate the following result −
10 -eq 20: a is not equal to b 10 -ne 20: a is not equal to b 10 -gt 20: a is not greater than b 10 -lt 20: a is less than b 10 -ge 20: a is not greater or equal to b 10 -le 20: a is less or equal to b
The following points need to be considered while working with relational operators −
There must be spaces between the operators and the expressions. For example, 2+2 is not correct; it should be written as 2 + 2.
if...then...else...fi statement is a decision-making statement which has been explained in the next chapter.