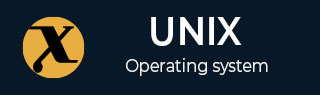
- Unix / Linux - Home
- Unix / Linux - What is Linux?
- Unix / Linux - Getting Started
- Unix / Linux - File Management
- Unix / Linux - Directories
- Unix / Linux - File Permission
- Unix / Linux - Environment
- Unix / Linux - Basic Utilities
- Unix / Linux - Pipes & Filters
- Unix / Linux - Processes
- Unix / Linux - Communication
- Unix / Linux - The vi Editor
- Unix / Linux - Shell Scripting
- Unix / Linux - What is Shell?
- Unix / Linux - Using Variables
- Unix / Linux - Special Variables
- Unix / Linux - Using Arrays
- Unix / Linux - Basic Operators
- Unix / Linux - Decision Making
- Unix / Linux - Shell Loops
- Unix / Linux - Loop Control
- Unix / Linux - Shell Substitutions
- Unix / Linux - Quoting Mechanisms
- Unix / Linux - IO Redirections
- Unix / Linux - Shell Functions
- Unix / Linux - Manpage Help
- Advanced Unix / Linux
- Unix / Linux - Standard I/O Streams
- Unix / Linux - File Links
- Unix / Linux - Regular Expressions
- Unix / Linux - File System Basics
- Unix / Linux - User Administration
- Unix / Linux - System Performance
- Unix / Linux - System Logging
- Unix / Linux - Signals and Traps
Unix / Linux - Shell String Operators Example
The following string operators are supported by Bourne Shell.
Assume variable a holds "abc" and variable b holds "efg" then −
Operator | Description | Example |
---|---|---|
= | Checks if the value of two operands are equal or not; if yes, then the condition becomes true. | [ $a = $b ] is not true. |
!= | Checks if the value of two operands are equal or not; if values are not equal then the condition becomes true. | [ $a != $b ] is true. |
-z | Checks if the given string operand size is zero; if it is zero length, then it returns true. | [ -z $a ] is not true. |
-n | Checks if the given string operand size is non-zero; if it is nonzero length, then it returns true. | [ -n $a ] is not false. |
str | Checks if str is not the empty string; if it is empty, then it returns false. | [ $a ] is not false. |
Example
Here is an example which uses all the string operators −
#!/bin/sh a="abc" b="efg" if [ $a = $b ] then echo "$a = $b : a is equal to b" else echo "$a = $b: a is not equal to b" fi if [ $a != $b ] then echo "$a != $b : a is not equal to b" else echo "$a != $b: a is equal to b" fi if [ -z $a ] then echo "-z $a : string length is zero" else echo "-z $a : string length is not zero" fi if [ -n $a ] then echo "-n $a : string length is not zero" else echo "-n $a : string length is zero" fi if [ $a ] then echo "$a : string is not empty" else echo "$a : string is empty" fi
The above script will generate the following result −
abc = efg: a is not equal to b abc != efg : a is not equal to b -z abc : string length is not zero -n abc : string length is not zero abc : string is not empty
The following points need to be considered while using the operator −
There must be spaces between the operators and the expressions. For example, 2+2 is not correct. It should be written as 2 + 2.
if...then...else...fi statement is a decision-making statement which has been explained in the next chapter.
unix-basic-operators.htm
Advertisements